Today, we will learn some more validation or pattern matching which many websites include in their login page (or anywhere according to their need). These patterns include Date of Birth matching, PAN Card matching, matching of some social security number such as Aadhaar Card, Validation of Credit or Debit Card Number, etc. Now, these are some sensitive information so before writing forward, I want to put a disclaimer here:
Disclaimer: Any number used in this post as PAN Card Number, Aadhaar Card Number or Debit Card Number is only for learning validation. It’s imaginary in my knowledge. Still if it’s real and exists and allotted to someone, it’s a shear coincidence.
Let’s begin with validating Aadhaar Card Number. Aadhaar Card is an unique number issued to every citizen of India. It is used as an ID Proof, as an address proof and it’s mandatory for opening bank account. Therefore, validating Aadhaar Card is very important.
#Task 1 (Day 6): Write a program to validate Aadhar Card Number.
What should be the approach:
- Enter Aadhaar Card Number.
- Aadhaar Number is a 12 digit number, which is written as 3 set of 4 digits separated by a ‘ – ‘ or ‘ / ‘ or simply space. For example: 1234/5678/4321 or 1234-5678-4321. This we have studied.
- 1st we have to check the length. len() will help. The length of entered numbers should be 12.
- \d will solve our purpose. We can write \d{4} to represent digits 4 times. Or we can also write \d\d\d\d.
- Print the validation successful message if everything is correct or an error message if pattern matching fails.
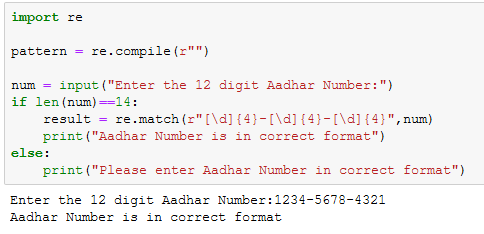
The code is self explainatory as written in algorithm above. Only important thing is 4th line of code. Length is 14. 2 extra characters are required to denote space, ‘ – ‘ or ‘ / ‘. Using length 12 will not allow to insert any character in between,
#Task 2 (Day 6): Write a program to validate Date of Birth.
DoB is another important parameter which is used in many login pages, such as, e-filling of Income Tax Department. DoB validation is almost similar to our Task 1 written above. The only difference is length of digits. The format which is followed in India mostly is dd-mm-yyyy. \d{2}-\d{2}-\d{4} will solve our purpose. Also, the overall length has to be 10 (including spaces).

It’s working correctly. Again, there can be many formats, depending on country to country and website to website. We can have mm-dd-yyyy or mm-dd-yy. Accordingly, 5th line of code can change. Moving to another task, let’s validate PAN Card.
#Task 3 (Day 6): Write a program to validate PAN Card number.
PAN Card is Personal Account Number Card. It’s an important card requires for filing tax, opening account etc. Income Tax Department use PAN Number as its login ID while logging in to their website. What should be our approach?
- PAN number is a 10 digit number (without space) and includes alphanumeric characters.
- 5 characters followed by 4 digits and 1 character at last.
- The code has to be like the above code, except line 5, which can be written as \D{5}\d{4}\D{1}. \D is used for non-digits as we have studied. Will it solve our purpose?
- Also, we have to check that it starts and ends with a non digit character. Anchors ^ and $ will help.

We can also write [A-Z] in place of \D. Both have the same meaning. Infact, [A-Z] is abetter approach because PAN is generally written in uppercase and \D supports both upper and lower case.

Checking for [A-Z],

This brings to our final task of Day 6. Validating a Debit Card or Credit Card Number. These are generally used by merchandise websites where you have to enter your card number to make payments.
#Task 4 (Day 6): Write a program to validate Debit Card Number.
The task is exactly similar to our today’s Task 1. There are 16 digits usually in a debit or credit card (in India atleast), arranged in 4 sets of 4 digits separated by space. I think, we can easily do this one.

There are 16 digits and 3 positions are required to insert any form of separator, either / or – or space. So, the length of entered number has to be 19. One final run of code by inserting space,
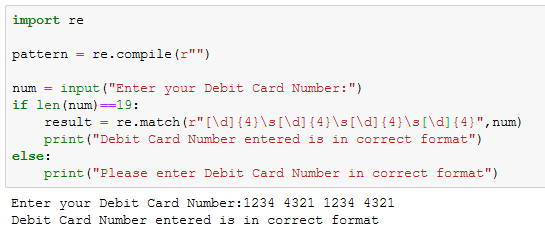
\s is used to insert spaces in between two consecutive characters. Here, \d{4}\s\d{4} will allow the user to separate 4th and 5th character with a space while entering the card number. This is important to know because simply writing \d (space) \d will not let you to insert space, like \d-\d or \d / \d let you to insert – or /.
So, today we learned few miscellaneous validation codes which are (and are not sometimes) essential. This concludes our Day 6 also. Thank You.